The data structure is a way of organizing data in computers to reduce complexity of problems and programs to use them effectively.
The data structure provides a means of efficiently organizing, managing, and storing data. It also includes data collection as well as operations that indicate how the flow of data should be controlled and how a data structure should be designed and implemented to reduce the complexity and increase the efficiency of an algorithm.
The theory of structure not only introduces data structures, but also helps you to understand and apply the concept of problem abstraction analysis step by step and to develop a good algorithm to solve the real problems.
The data structures help you to understand the relationship of the data elements with their order.
Data structures are used in almost all areas of computer science and programming, database design and from operating systems to front-end development to machine learning. E.g. Array, Linked Lists, Stack, Queues, Trees, Graphs, Sets, Hash Tables.
Types of Data Structure:
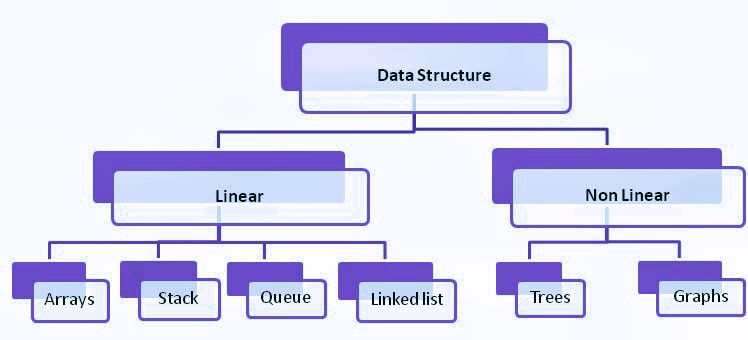
Linear Data Structures:
The linear data structure is one of the types of data structures in which data is stored and accessed sequentially, however, the elements can be stored in these data structures in any order.
Examples of linear data structures are array, linked list, stack, queue, hashing, etc.
Array:
Array is a collection of similar data types. Arrays are beautiful because of their simplicity and are useful in situations where the number of data elements is known (or can be determined programmatically).

Uses:
- Database entries are generally implemented as arrays.
- Used in computer to maintain lookup tables.
Linked List:
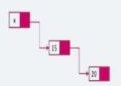
A linked list is a collection of nodes. Linked lists have advantages over arrays. It takes less space as compare to array. Size depends upon the nodes.
Linked lists have advantages over arrays, in the array searching operation, the operation requires O (1) time complexity, but insert and remove require O (n) time complexity, which is a bit costly, but these linked list operations are fast.
Uses:
- Message delivery on network (message is broken into packets and each packet has a key of the next one so that at the receiver’s end , it will be easy to club them)
- Browser cache which allows to use back button.
Stack:
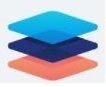
Stack is LIFO (Last in First Out) data structure where the element that added last will deleted first. There are some basic operations that are performed on the stack, such as push(), pop(), top(), isempty(), etc. The stack has a variety of uses.
Uses:
- Browsers use stack data structure to keep track of the last visited sites.
- Call log in mobiles use stack.
Queue:
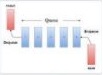
Queue is FIFO (First In First Out) data structure where the element that added first will delete first.
In real life, priority is given according to whoever comes first, and the queue in computer science has the same meaning. The queue also has the same operations only defined for stack instead of top (), the queue has Front () and Rear () which helps us to get the first and last data from the queue.
Uses:
- To implement printer spooler so that jobs can be printed in the order of their arrival.
- CPU task scheduling.
- In real life scenario, Call Center phone systems uses Queues to hold people calling them in an order, until a service representative is free.
Non-Linear Data Structures:
In non-linear data structures, elements are not stored linearly. The data elements have a hierarchical relationship that includes the relationship between child, parent, and grandparent. Such data structures support multi-tier storage and sometimes cannot be traversed. These data structures are not easy to implement, but they are more efficient in memory utilization as compared to the linear data structures.
The most common non-linear data structures are trees, binary search trees, graphs, heaps, tries, segment trees, etc.
TREE:
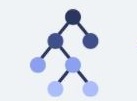
In trees, data is stored and accessed hierarchically. The tree is a multilevel data structure defined as a set of nodes, with the top node being referred to as the root node while the bottom-most nodes are referred to as the leaf nodes.
Each node has only one parent node but can have multiple children nodes
Types of Tree in Data structure:
- General Tree
- Binary Tree
- Binary Search Tree
- AVL Tree
- Red Black Tree
- N-ary Tree
Uses:
- To store the genealogy information of biological species.
- The database also uses a tree data structure for indexing.
- File System – be it NTFS or be it EXT2 all of them stores file metadata as trees.
Graph:
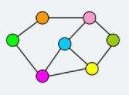