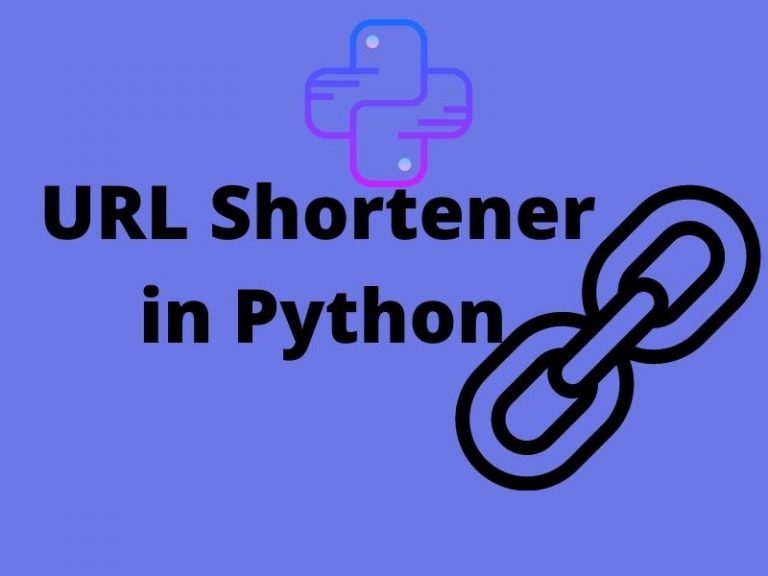
In this blog post, you will learn how to use the pyshorteners module to create a URL shortener. Pyshorteners is a famous Python library that helps to short and expand URLs using the most famous URL Shorteners available like Adf.ly, Bit.ly, Chilp.it, Clck.ru, Cutt.ly, Short.cm and Tiny.cc, etc. URL shortening is the process to reduce the length of the URL and making them easier to share. There are many messaging platforms that have limits on the length of the message. Here anyone can face the problem of sharing the long URLs so that they can be easily shared on platforms like Twitter where the number of characters is a problem and it looks nice when some URLs have a short length. There are so many URL shorteners out there today that we’re going to be implementing them in Python.
How does a URL Shorting tool works?
The URL Shortening Tool accepts the URL of a page and provides you with a shortened version of this URL by using some algorithms and short both the long and short URL in their database. The short URL does not contain the actual URL text details but provides you with the URL of the domain name of the URL shortening tool with the redirect ID like https://www.domainname. com/short_url_id in which that id can be of any length but in most cases, it is seven-character long in short URLs. The short message you receive will redirect you to the real URL by checking in their database which is mapped to that short URL. This means that you can use the short URL as an alternative to the real URL.
Installation:
pip install pyshorteners
Code to create short URL using tinyurl:
import pyshorteners
#inout long URL
link_input = input('What link would you like to shorten?\n')
link = link_input
#initialize the Shortener
shortener = pyshorteners.Shortener()
#short the url with tinyurl
url = shortener.tinyurl.short(link)
#print short url
print(url)
OUTPUT:

Code to create short URL using tinyurl with Graphical User Interface:
from tkinter import *
import pyperclip
import pyshorteners
def url_shortner():
shortener = pyshorteners.Shortener()
url_short = shortener.tinyurl.short(main_url.get())
#set the gloabal short_url
short_url.set(url_short)
def copy_url():
#copy short url on clipboard
pyperclip.copy( short_url.get())
if __name__=="__main__":
root = Tk()
root.geometry("700x700")
root.title("My URL Shortener App")
root.configure(bg="#34568B")
main_url = StringVar()
short_url= StringVar()
Label(root, text="Enter The Main URL", font="Times").pack(pady=5)
Entry(root,textvariable=main_url, width =100).pack(pady=5)
Button(root, text="Generate Short URL", command =url_shortner).pack(pady=5)
Label(root, text="The Short URL ", font="Times").pack(pady=5)
Entry(root, textvariable= short_url, width=50).pack(pady=4)
Button(root, text="Copy the Short URL", command= copy_url).pack(pady=5)
root.mainloop()
OUTPUT:
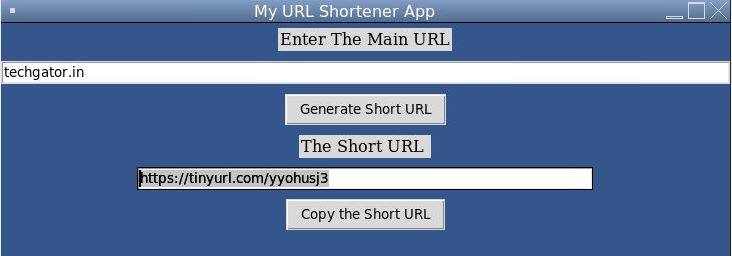