Asynchronous JavaScript and XML (AJAX) is a web development technique used to create dynamic and responsive web pages without reloading the entire page. AJAX allows a website to retrieve and display new data without interrupting the user’s interaction with the website. This technique was first introduced in 2005 by Jesse James Garrett and quickly became a popular method for developing web applications.
How AJAX Works?
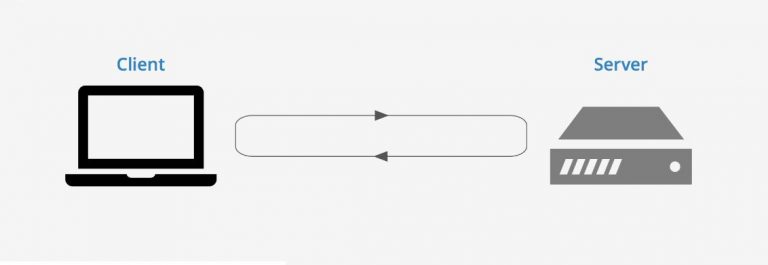
AJAX uses a combination of JavaScript and XML to communicate with the server without reloading the entire page. When a user interacts with a web page, the browser sends a request to the server using an XMLHttpRequest (XHR) object. The server then responds to the request with the necessary data, which is typically in XML or JSON format. The JavaScript code in the web page then updates the page with the new data, without the need for a page reload.
Examples of AJAX:
Here are some examples of AJAX in action:
- Autocomplete Search: One common use of AJAX is for autocomplete search. When a user types in a search query, the web page can use AJAX to send a request to the server to retrieve matching results. The server responds with the matching results, which the JavaScript code then displays in a dropdown menu. This allows the user to quickly find what they are looking for without having to navigate away from the page.
- Infinite Scrolling: Infinite scrolling is another example of AJAX in action. Instead of loading all of the content on a page at once, the web page can use AJAX to load new content as the user scrolls down the page. When the user reaches the bottom of the page, the JavaScript code sends a request to the server to retrieve the next set of content, which is then added to the page. This creates a seamless and uninterrupted browsing experience for the user.
- Form Submission: AJAX can also be used for form submission. When a user submits a form, the JavaScript code in the web page can use AJAX to send the form data to the server without reloading the entire page. The server then responds with a success or error message, which the JavaScript code can display on the page. This allows the user to submit a form without losing any data or having to navigate away from the page.
Example of how to fetch data using AJAX in HTML
AJAX Example
Joke Generator